Update: Jan. 11, 2012
This script has been used as the basis of a Modernizr plugin by Jasper Palfree.
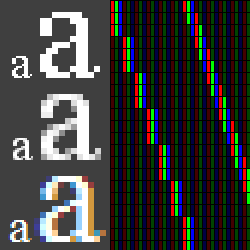
In an earlier article, I mentioned that Boing-Boing had a few issues when they wanted to use @font-face embedding inside their website. In short, the problem was that some fonts look bad on computer monitors without font-smoothing enabled in the operating system. This brought on a lot of discussion as to whether there was a way to detect whether or not font-smoothing was being used using JavaScript. I initially thought there wasn’t a way, but after seeing a promising but incomplete method of detecting font-smoothing, I spent a few days devising a way to do it.
Internet Explorer Does Something Easily (For a Change)
Paul Irish mentioned to me and a few other colleagues that he came across a page using an Active X control that detects font-smoothing in IE. I was so hopeful … until I realized that this only works on browsers that have come into contact with Microsoft’s online Cleartype Tuner, which I visited with my copy of IE months before. If a users had never visited this page, the script would fail.
I was more disappointed because Googling “javascript cleartype” did not point to anything useful. However, searching for “javascript font-smoothing” pointed me to an article that told me about Internet Explorer’s screen.fontSmoothingEnabled property. This gives us what we need … but only in Internet Explorer. How on Earth can we detect font-smoothing in other browsers, and in non-Windows operating systems?
Canvas to the Rescue
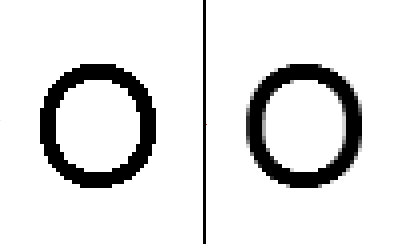
Letter 'O' , Arial font, 32 px, rendered both without (left) and with (right) font-smoothing
I then thought about about the screenshots I made for the @font-face in Depth article. Can a browser render a black glyph and detect if there is some sort of non-black pixel colouring around the its edges of the glyphs? A human can tell the difference: if there are some non-black pixels around the edge of the glyph, it must be using font-smoothing.
But web browsers can do this too! Just have the browser draw a letter in black inside a canvas
tag, and then have it sift through the canvas’ pixels to see if there are any that are not pure black or pure white (more accurately, have the browser check the alpha channel to see if there are any semi-transparent pixels, which have a value that is not 0 or 255). If there are no semi-transparent pixels, then the algorithm assumes that no font-smoothing is being used. I wrote an JavaScript routine that does this – it starts from co-ordinate (8,1) and scans left to right, to the bottom of the canvas (any point on near the top on the left-hand side of the canvas would have done as well).
The result is a JavaScript object, TypeHelpers
, which implements this routine in one method, hasSmoothing()
:
var TypeHelpers = new function(){ // I use me instead of this. For reasons why, please read: // http://w3future.com/html/stories/callbacks.xml var me = this; me.hasSmoothing = function(){ // IE has screen.fontSmoothingEnabled - sweet! if (typeof(screen.fontSmoothingEnabled) != "undefined") { return screen.fontSmoothingEnabled; } else { try { // Create a 35x35 Canvas block. var canvasNode = document.createElement('canvas'); canvasNode.width = "35"; canvasNode.height = "35" // We must put this node into the body, otherwise // Safari Windows does not report correctly. canvasNode.style.display = 'none'; document.body.appendChild(canvasNode); var ctx = canvasNode.getContext('2d'); // draw a black letter 'O', 32px Arial. ctx.textBaseline = "top"; ctx.font = "32px Arial"; ctx.fillStyle = "black"; ctx.strokeStyle = "black"; ctx.fillText("O", 0, 0); // start at (8,1) and search the canvas from left to right, // top to bottom to see if we can find a non-black pixel. If // so we return true. for (var j = 8; j <= 32; j++) { for (var i = 1; i <= 32; i++) { var imageData = ctx.getImageData(i, j, 1, 1).data; var alpha = imageData[3]; if (alpha != 255 && alpha != 0) { return true; // font-smoothing must be on. } } } // didn't find any non-black pixels - return false. return false; } catch (ex) { // Something went wrong (for example, Opera cannot use the // canvas fillText() method. Return null (unknown). return null; } } } me.insertClasses = function(){ var result = me.hasSmoothing(); var htmlNode = document.getElementsByTagName('html')[0]; if (result == true) { htmlNode.className += " hasFontSmoothing-true"; } else if (result == false) { htmlNode.className += " hasFontSmoothing-false"; } else { // result == null htmlNode.className += " hasFontSmoothing-unknown"; } } } // if EventHelpers.js is included, insert the hasFontSmoothing CSS classes if (window.EventHelpers) { EventHelpers.addPageLoadEvent('TypeHelpers.insertClasses') }
Note the object also has an insertClasses()
method. This method, when run, adds a class to the html
tag:
hasFontSmoothing-true
if font-smoothing is being usedhasFontSmoothing-false
if it is nothasFontSmoothing-unknown
if the user agent is unable to tell
This makes it easy for developers who don’t want to mess with JavaScript code and just want to use CSS.
Also note the EventHelpers.addPageLoadEvent()
call at the end of the code. This method (which is part of EventHelpers.js
, included with the archive below) implements Dean Edwards’ window.onload alternative which doesn’t wait for all the objects in the page to be loaded. I use this implementation to execute TypeHelpers.insertClasses()
when the page loads so any font-detection CSS rules will work right away. Please feel free to change this code to use the equivalent function call in Dojo, Prototype, jQuery, or whatever JavaScript code framework you prefer.
Example #1: JavaScript Font Smoothing Detection
Enough of theory … let’s look at it in practice! To show how to detect font-smoothing with JavaScript, I created a page that, when the page is loaded, checks to see if it can tell if font-smoothing has been implemented and tells the user. Here is the code that does this check:
function displayInfo() { var message; var isFontSmoothingOn = TypeHelpers.hasSmoothing(); if (isFontSmoothingOn == true) { message = "This browser is using a font-smoothing technology"; } else if (isFontSmoothingOn == false) { message = "This browser isn't using a font-smoothing technology" } else { message = "We could not detect if font-smoothing is being used." } document.getElementById('detectInfo').innerHTML = message; } window.onload = displayInfo;
Example #2: CSS Font Smoothing Detection
As implied earlier, this library can help CSS use different fonts if the browser is using a font-smoothing technology. For example, using the following CSS will allow a browser to use the Droid Sans embedded font only if it using font-smoothing — otherwise, it will use Arial:
@font-face { font-family: "Droid Sans"; src: url("/shared/fonts/DroidSans/DroidSans.eot"); src: local("Droid Sans"), local("Droid Sans"), url("/shared/fonts/DroidSans/DroidSans.ttf") format("truetype"); } body { font-family: "Arial", "Helvetica", sans-serif; } html.hasFontSmoothing-true body { font-family: "Droid Sans", "Arial", "Helvetica", sans-serif; }
We can also serve special content to users depending on the way fonts are rendered on their browser. We first create content for all three scenerios (browser uses font-smoothing, browser doesn’t use font-smoothing, and the “we cannot detect” case) and wrap the content inside <code>div</code> tags using appropriate CSS classes:
<div class="fontSmoothingMessage initiallyHidden"> <p>You browser <strong>is</strong> rendering this page with font-smoothing. Because of that, we will attempt to serve up the Droid Sans font to render this page, because we think it looks cool. If you are using a browser (such as Google Chrome) that cannot render downloaded True Type fonts by default, then the page will be rendered using Arial instead.</p> </div> <div class="noFontSmoothingMessage initiallyHidden"> Your browser <strong>is not</strong> rendering this page with font-smoothing. It is for that reason we have decided to use the plain old Arial font to render this page, because it is hinted for use for displays that don't employ a font-smoothing technology. </div> <div class="unknownFontSmoothingMessage initiallyHidden"> <strong>We are not sure</strong> if your browser is rendering this page with a font-smoothing technology. It is for that reason we have decided to use the plain old Arial font to render this page, because it is hinted for use for displays that don't employ a font-smoothing technology. </div>
Note all the div
tags are members of the class initiallyHidden
. This class will be used to hide all font-smoothing related content until the script kicks in.
However, all this will not work unless we use the following CSS code:
.initiallyHidden { display: none; } html.hasFontSmoothing-true .fontSmoothingMessage, html.hasFontSmoothing-false .noFontSmoothingMessage, html.hasFontSmoothing-unknown .unknownFontSmoothingMessage { display: block; }
Of course, this whole soltution relies on whether JavaScript being turned on in the user’s browser. This should be kept in mind when implementing this solution.
Download
All code used in this article can be downloaded below (Note: version 1.0 was missing the Droid Sans Fonts which have now been put into the archive. Thanks to John Faulds for pointing this out).
TypeHelpers.js v.1.0a and sample code.
With the help of Tim Brown, it was determined that the code detected font-smoothing correctly in the following browsers:
- Internet Explorer 6 and up on Windows XP and higher.
- Firefox 3.5 and higher on Windows XP and higher, Mac OS X 10.4 and higher, and Ubuntu Linux 9.10 (and probably lower)
- Chrome 3.0 on Windows XP and higher
- Safari 4.0.3 on Windows XP and higher and Mac OS X 10.4 and higher
This script cannot detect font-smoothing in any version of Opera (at the time of this writing, this includes all versions up to 10.10), since it cannot write text inside the canvas element in a way we can poll the pixels afterwards. If anyone can find a way of making it work with Opera, please write a comment below — I’d love to be able to support this browser.
Testing Caveats
Testing font-smoothing in most Windows web browsers is easy since it can be turned off inside the Display control panel. However, when using Safari for Windows, it is necessary to navigate inside Safari’s Appearance preferences and set the Font-smoothing option to Windows Standard. This is because by default, Safari uses it’s own built-in font-rendering engine which doesn’t seem to render aliased fonts. In Mac OS X, it seems anti-aliasing only works for fonts below a certain size, so aliased fonts don’t seem to be an issue with that operating system. In Ubuntu Linux I have yet to find a way of shutting of font-smoothing. If anyone knows a way, please let me know.
74 responses so far
1
Paul Irish
// Nov 29, 2009 at 4:11 pm
The canvas hack is righteous. Really nice work, Zoltan.
In Win XP (not sure about vista/7), there are two smoothing options: Standard and Cleartype.
The Active X approach would have identified ClearType in particular, but this approach seems to give a positive result with either choice, correct? I’d bet 90% of people with Font Smoothing enabled are using ClearType, so this distinction is mostly moot.
2
zoltan
// Nov 29, 2009 at 4:30 pm
Paul: you are right – either ClearType or Standard font-smoothing will give a positive result. I believe that you are right that most Windows XP and above users use ClearType – I would love to see if there were any stats on that though.
3
Philip
// Nov 29, 2009 at 7:05 pm
Great idea!
One suggestion: In your Droid/Arial example, I’d change the CSS to let @font-face be the default, and switch to Arial only when smoothing is *not* enabled. My guess is most people will have smoothing enabled. Forcing them to wait for JS to execute will delay loading of @font-face, increasing the likelihood of seeing that dreaded flash of unstyled text when initially loading the font.
4
zoltan
// Nov 30, 2009 at 12:17 am
Philip: that is a great point – it’s what I was thinking when I was first drawing up that example, since I don’t like taking away functionality to JavaScript-disabled browsers if I can help it.
The problem was that sometimes, the script would kick in after the Droid font would load, causing the user to first see the Arial font, then the loaded Droid font, and then back to the Arial font again.
The question is: for the amount of users that this affects, is it really an issue, and should we have Droid load by default? I’m not sure. Maybe the right solution is to do a combination of this script and Paul Irish’s Anti-@font-face-FOUT script.
5
Dainis Graveris
// Nov 30, 2009 at 2:49 am
Hah, great tip, I didn’t know such effects can be achieved with Javascript! Fresh information for me, thanks!
6
Aaron Peters
// Nov 30, 2009 at 6:02 am
Zoltan,
this is *great stuff* !!!
FYI, anti-FOUT can be accomplished by ‘flushing the document early’.
Article based on first, basic testing is here: http://www.aaronpeters.nl/sandbox/flush-font-face
– Aaron
7
Richard Fink
// Nov 30, 2009 at 10:54 am
Zoltan,
Yes both Cleartype and Standard return positive in XP.
Clever script – BTW. Maybe someday browser makers will give scripters all the methods, properties, and events we need. Until then, we query for the info indirectly. I look at it like paying taxes.
Be aware that in FF 3.6 a new ability to smooth images in the Canvas tag is appearing.
Don’t know how that might impact this work. Just mentioning it.
rich
8
zoltan
// Nov 30, 2009 at 11:27 am
Richard: thanks for the tip regarding Firefox 3.6. Will be looking into it for sure and updating if needed.
9
John Faulds
// Nov 30, 2009 at 6:00 pm
Just a heads-up that the cssExample.html in the zip references DroidSans fonts in the shared folder that aren’t actually included.
10
Dustin Wilson
// Nov 30, 2009 at 6:14 pm
After reading this I initially thought I could get around Opera’s lack of support for the canvas text API, but it doesn’t work either. Opera can draw SVG images to the canvas, so I thought I could just write an SVG image containing a rendering of the letter O in 32px Arial then pixel manipulate that. It wouldn’t work because of security violations for multiple reasons. One is that images whose source are Data URIs can’t be manipulated in the canvas. Additionally for some stupid reason SVG can’t be manipulated either, even using toDataURL(). Guess there’ll have to be a wait for Opera’s support for the canvas text API.
11
zoltan
// Nov 30, 2009 at 11:11 pm
John: Thanks Dude! I updated the zip file to include the fonts … something I missed during my testing. Many thanks.
12
Ace
// Dec 1, 2009 at 3:44 am
Thanks for doing the research on this issue, zoltan. This’ll bring us closer to unifying the way fonts are displayed across browsers and operating systems. These inconsistencies are the main reason why I don’t to make extensive use of custom fonts on websites. I made an attempt a while ago, but I was heavily disappointed after comparing the rendering between Windows and Mac, and I’m not even mentioning Linux (all using the same browser, Firefox).
13
zoltan
// Dec 1, 2009 at 8:41 am
Ace: it is for these reasons why we are promoting the Type Rendering Project! We hope to make this into a forum and discussion on how to make fonts look good on the web now. Hopefully this article is a good start in that direction.
16
Agos
// Jan 30, 2010 at 7:16 am
Testing it on the iPhone yelds negative results, but AFAIK subpixel AA is always active on the iPhone.
17
zoltan
// Jan 30, 2010 at 6:26 pm
@Agos: That’s intreresting. That would imply that either the iphone’s browser doesn’t support canvas, or that that fonts in canvas don’t render the same as in plain HTML. I’ll have to see if I can test on a friend’s iphone and update the routine. Thanks for letting me know – I’ll let you know when there is a fix.
19
Matt Stow
// Feb 3, 2010 at 11:20 pm
It would be good if this could detect whether ClearType was being used as opposed to just standard font smoothing too.
20
zoltan
// Feb 4, 2010 at 12:37 am
Paul Irish told me about an Active X control that can do just that, but we discovered it wasn’t on every IE install. I don’t believe there is any way to detect a specific font-smoothing technology in the other browsers. :-/
22
teoulas
// Apr 8, 2010 at 5:24 pm
Nice one.
Here’s how to disable font anti-aliasing in Gnome, if you haven’t found out yet:
Go to System > Preferences > Appearance. Click ‘Fonts’ and then select ‘Monochrome’ rendering. For more control over font rendering click the ‘Details…’ button.
Screenshot: http://img85.imageshack.us/img85/4710/screenshotappearancepre.png
23
Sven
// May 11, 2010 at 4:56 am
I am absolutely loving this solution!
Many thanks :)
24
Lars G. Sehested
// Jul 19, 2010 at 10:15 am
@Matt Stow: If you change “32px Arial” to e.g. “12px Arial” in line 55 of TypeHelpers, it will only set “hasFontSmoothing-true” if ClearType is enabled. I suppose it would be easy to run a test at each font size to distinguish between none, Standard or ClearType.
25
James
// Sep 8, 2010 at 4:59 am
Great solution. Many thanks dude :-)
26
Ric
// Sep 28, 2010 at 5:21 pm
Pure genius!
28
Reimund Trost
// Oct 20, 2010 at 5:01 pm
Awesome work Zoltan. This will come in really handy for my site which suffer from many Internet Explorer / Win XP users!
29
Andrew Barr Brunger
// Nov 6, 2010 at 1:21 pm
Fantastic solution. Many thanks!
I did not feel comfortable serving non-system font based on presumptions about a users browser and OS. Your script works flawlessly and is incredibly important.
Andrew Barr Brunger
30
Robine
// Nov 16, 2010 at 3:31 am
This looks like something I should keep in mind. Currently I am using sIFR, but I will definitly give this a go. If I encounter something useful, I will let you know. Thanks for the time you took to figure this out!
31
Michael van Laar
// Nov 16, 2010 at 3:34 am
Great article and even greater script. I recently combined the neccessary parts (at least as far as my rather uncomplete JavaScript knowledge allowed me to tell what was necessary) of EventHelpers.js with TypeHelpers.js and Paul Irish’s FOUT fighting script. The result is a 3 KB (only 1.3 KB with gzip) big JavaScript file which handles all of my Webfont loading problems. I use this combined script it on all my websites. (combined-script download and information here: http://www.michael-van-laar.de/blog/artikel/webfont-load-enhancer/ – Website only in German, readme.txt inside the zip file in English) Works great!
Of course I also included Lars G. Sehested’s “12px Arial” configuration tweak in the documentation of my combined script. You both are credited various times, so I hope my “set it and forget it” script solution is OK for you.
33
zoltan
// Nov 16, 2010 at 8:39 am
@Michael: I am glad you find my work (and Paul’s) of use. Remixes are more than welcome! If you make any additions or changes, please let me know.
34
Matthew Lein
// Nov 23, 2010 at 8:30 am
I can verify that on the iPad, TypeHelpers.hasSmoothing() spits out false. :(
I’ve seen that the canvas handling is definitely a little funky from my previous experiments, but I haven’t dug into why/fixes. I’ll let you know if I come up with anything.
35
Matthew Lein
// Nov 23, 2010 at 8:30 am
Update: iOS 4.2 fixes the detection. So its not much of an issue.
38
Ian
// Dec 27, 2010 at 3:52 pm
Perhaps some of you are aware that there are obvious differences in font rendering between standard and cleartype methods on XP. I use webfonts on a site that drives photo portofolios and these fonts anti alias perfectly when windows is using the standard method (antialiasing is monochrome in this case) Cleartype however(which is enabled by default in IE8, regardless of the system setting) however smooths most of these fonts in an uglier, jagged way, especially ar larger sizes :( I think Cleartype is default in Windows 7, and XP has as default the Standard method. I would rather use cleartype fonts, such as Corbel or Calibri if cleartype is enabled that a webfont that ends up looking worse.
41
Jos Hirth
// Feb 15, 2011 at 6:29 am
>That would imply that […] fonts in canvas don’t render the same as in plain HTML.
Regular text rendering will typically use subpixel rendering (ClearType and the like), but that won’t work inside a Canvas. Subpixel rendering isn’t a very good idea if the result might be transformed.
>ctx.getImageData(i, j, 1, 1)
It should be faster if you grab all 800 pixels at once. Even if you only check a few of them. The call itself is pretty expensive and the data doesn’t have much weight (relatively). Sorta like a cruiser ship with only one passenger. Doesn’t really matter if there are more of them.
42
Ira F. Cummings
// Feb 18, 2011 at 2:16 pm
This seems to be a great solution for me, but I’m curious about some details. I’m serving FF Meta to a site using Typekit, and it’s been looking horrible—near unreadable—on PC’s with font smoothing disabled. I’ve implemented your script, so I’m off to test it.
BUT in my research for this issue, some people have stated that IE doesn’t recognize the canvas. See here for the clearest discussion: http://www.typophile.com/node/77635
Is there any truth to this? Can your method detect font smoothing with IE?
Lastly, could you explain or provide a suggestion on how I would use jQuery instead of the EventHelpers.js? I’m a javascript newb, so excuse my ignorance, but I’m using this on a site that is already loading jQuery.
Thanks!
43
zoltan
// Feb 19, 2011 at 1:52 am
@Ira: The script first checks to see if
screen.fontSmoothingEnabled
is present (which is true only in IE). If it is present, it uses it to see if font-smoothing is on. If not, then it does the canvas check.screen.fontSmoothingEnabled
is true if ClearType or “Standard” smoothing is on, so it should be a bulletproof test.As for using jQuery instead of EventHelpers, this should be quite easily done. Just take out the
if
condition at the end of TypeHelpers.js and replace it with a call to$.ready()
. This article should help a little.44
zoltan
// Feb 19, 2011 at 2:14 am
@Jos: I am unsure about what you meant about your first comment, could you clarify? This algorithm does work in all the four canvas enabled desktop browsers, so I am not sure as to what you mean — I must be missing something.
As for the second comment, I was unaware how expensive the
.getImageData()
call is .. thanks for the tip. Will have to update this in a future release.46
Ira F. Cummings
// Mar 4, 2011 at 11:25 am
Thanks a lot. Really appreciated.
Still not sure about the jQuery solution, mostly because I’m not sure what EventHelpers is doing…and how to make jQuery do the same thing. I know how to use jQuery to get basic things done, it’s the more complicated stuff that I’m not sure about how to use it in this situation. I won’t bug you with it though…see if I can figure it out with some web sleuthing.
47
matthewlein
// Mar 4, 2011 at 11:46 am
FYI Zoltan, the @Jos comment#2 is exactly what I sent you months ago…
If you get all 800 pixels its only faster for browsers that fail, but your current method of getting pixels 1 at a time is faster for browsers that pass (which is now most browsers). This happens because to get find a semi-transparent pixel, you only need to make a few calls before it finds one (around 7-8 I think, can’t remember).
storing all 800 pixels (times the 4 RGBA values) is pretty slow, and only makes up for the speed when you access that data many many times.
anyone interested in it can check my jsPerf:
http://jsperf.com/canvas-loops/3
48
zoltan
// Mar 5, 2011 at 3:59 pm
@matthewlein: thanks for posting the jsPerf. Really good at illustrating this visually.
52
andy
// Aug 19, 2011 at 6:54 am
Can’t you detect ClearType or subpixel-based hinting by detecting if there are different r/g/b values in a single pixel rather than simply a value between and ?
53
andy
// Aug 19, 2011 at 6:55 am
Oops. Last post got messed up.
Can’t you detect ClearType or subpixel-based hinting by detecting if there are different r/g/b values in a single pixel rather than simply a value between (0,0,0) and (255,255,255)?
54
zoltan
// Sep 2, 2011 at 8:10 am
@andy: It may be worth looking into this. The problem is there is a way to tune cleartype, so I can’t rely on “every Arial O has these colored pixels in the middle”. If you or anyone else has any ideas about how to work around this, I’m all ears.
56
T
// Sep 29, 2011 at 2:34 am
Hi, first of all thanks for that your fontSmoothing detection is very cool. i have tested it a little bit and have seeen document.body.appendChild(canvasNode); is no longer needed in safari (win). if you kill this line you can fire the whole script in your head section and you do not have to wait for “dom ready”.
57
Chris Hamm
// Oct 10, 2011 at 5:28 pm
The demo shown always says I am using font smoothing tech even with ClearType turned off. Is font smoothing and ClearType separate from each other?
Using Windows 7 and FF 7 as well as IE 8 and 9
58
zoltan
// Oct 12, 2011 at 7:16 pm
@Chris: ClearType is one of many types of anti-aliasing/font smoothing technologies out there and, as far as I know, only available on the Windows platform. In Windows XP, one can turn off font-smoothing completely, but in Windows Vista and Windows 7 it seems that one can only choose to use ClearType or “vanilla” font-smoothing. I believe this is because the fonts that come with Vista and higher (e.g. Consolas, Calibri, and a whole bunch of others that begin with the letter ‘C’) require font-smoothing or they look like crap.
59
Michael van Laar
// Mar 16, 2012 at 4:53 pm
I use the script for years now. It seems that since the last Google Chrome Update (Version: 17.0.963.79) the script has a problem with Chrome on Win7. It always comes to the (wrong) conclusion “hasFontSmoothing-false”. In IE 9 and FF everything works as a charm.
60
David Craig
// Apr 16, 2012 at 7:43 pm
I have just come across your script – getting frustrated at how other people’s websites look to me (with no font smoothing). However when I tried your real life example it reported that I have font smoothing on – and I don’t (at least I don’t think I do).
I am on Win7 x64 using Firefox 11. I have cleartype off and I have edited the registry values to remove all font smoothing: [HKEY_CURRENT_USER\Control Panel\Desktop] FontSmoothing = 0 and FontSmoothingType = 0
61
Alexander Kappler
// Apr 25, 2012 at 4:09 am
Hi Zoltan,
first of all, thank you for this wonderfull skript!
Since a few weeks, I do have the same Problem like Michael mentioned in the post before.
I was wondering whether you will provide an fix for that issue.
Thank you!
Alex
62
zoltan
// May 8, 2012 at 10:47 pm
@Alexander, @Michael: Sorry for the late reply — I must have missed these among all my other messages. When I check the script with Chrome 18, I don’t see any problems. Can you give me a URL to a page where this actually happens?
63
zoltan
// May 8, 2012 at 11:00 pm
@David: see my response to Chris’ comment above.
64
Michael van Laar
// May 17, 2012 at 9:31 am
@Zoltan: You’re right. The problems are gone in Chrome 18. Fine! I like problems which right themselves :-)
65
Gerrit van Aaken
// May 29, 2012 at 4:26 pm
Any ideas how to tell GDI rendering apart from DirectWrite rendering on Windows Vista oder Windows 7?
IE9 does only DirectWrite, Firefox 4+ does use DirectWrite in some cases, Chrome does not at all (yet).
You can visually detect DirectWrite by rotating text slightly: http://jsfiddle.net/Xq8yt/1/ (Only for Windows Vista/7: DirectWrite looks good, GDI looks horrible)
66
zoltan
// Jun 4, 2012 at 11:56 pm
@Gerrit: Not at this time, and I don’t see a simple solution to this. :-/
67
Kazi
// Jun 7, 2012 at 7:16 am
Excellent, this must be part of the modernizr feature detection javascript library. Why it isn’t?
68
zoltan
// Aug 12, 2012 at 11:13 am
@Kazi: a plugin for Modernizr has been created by Jasper Palfree.
70
ram almog
// Oct 8, 2012 at 4:55 am
Tip: If you are implementing this on a rtl html (right to left, like hebrew and arabic), note that you have to set the canvas to stay in ltr, or the letter you draw get out of bounds (just add canvasNode.style.direction = “ltr”; when creating the canvas.) Just spent an hour figuring this out…
71
zoltan
// Oct 15, 2012 at 8:29 pm
@ram: Thanks for the tip! Would not have known this — very useful to know. Much appreciated. :-)
72
Brandon Lebedev
// Jun 11, 2013 at 1:58 pm
This script returns a false positive in FF 21.0 on Win7 when system cleartype is off (the default).
Also, if users follow the method linked to below, this script will return a false positive in IE9 on Win7. Removing the `screen.fontSmoothingEnabled` test fixes the problem.
http://stackoverflow.com/questions/5427315/disable-cleartype-text-anti-aliasing-in-ie9#tab-top
73
zoltan
// Jun 16, 2013 at 12:36 pm
@Brandon: Unless things have changed, Cleartype is turned on by default it Windows 7, and therefore Firefox in Windows 7 as well.
The page you link to talks about how to go into the registry to disable ClearType. This is an edge case as the vast majority of users wouldn’t do (and would not know how to do) this.
74
Manish Salunke
// Oct 31, 2013 at 1:34 am
Ohh.. Great!! Thanks for sharing with us..
Give Feedback
Don't be shy! Give feedback and join the discussion.
Please Note: If you are asking for help using the information on this page or if you are reporting a bug with the code featured here, please include a URL that shows the problem you are experiencing along with the browser/version number/operating system combination where the issue manifests itself. Without this information, I may not be able to respond.